Cordova SQLite Storage Plugin
This Cordova/PhoneGap plugin uses SQLite databases on Android, iOS and Windows with an HTML5/Web SQL API.
Plugin ID/package name:
cordova-sqlite-storage
Tested version: 6.1.0
Demo
Import the SQLite storage plugin demo to your Monaca account
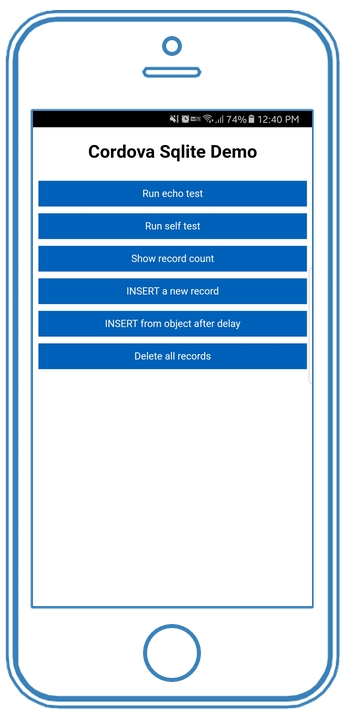
Enable the plugin in Monaca IDE
From the IDE menu, go to Config → Manage Cordova Plugins .
Click the Import Cordova Plugin button. Then, you can choose to import the plugin using a ZIP file or a URL/package name.
Usage
After importing the plugin into the project, you can initialize your database. Please make sure to call the plugin API after the Cordova is loaded.
var database = null;
document.addEventListener("deviceready", function(){
// Initialize the database after the Cordova is ready.
initDatabase();
});
function initDatabase() {
database = window.sqlitePlugin.openDatabase({name: 'sample.db', location: 'default'});
database.transaction(function(transaction) {
transaction.executeSql('CREATE TABLE SampleTable (name, score)');
});
}
API references
This section describes some of the main functions used in the Demo. For complete API references, please refer to the repository.
Self-test functions
echoTest()
Verify that both the Javascript and native part of this plugin are installed in your application.
window.sqlitePlugin.echoTest(successCallback, errorCallback);
Return Value
Promise
selfTest()
Verify that this plugin is able to open a database, execute the CRUD (create, read, update, and delete) operations, and clean it up properly.
window.sqlitePlugin.selfTest(successCallback, errorCallback);
Return Value
Promise
Opening a database
Open a database access handle object.
window.sqlitePlugin.openDatabase({name: String, [location: String], [iosDatabaseLocation: String]}, [successCallback], [errorCallback]);
Parameter
Name
Type
Description
name
String
Name of the database
location
String
[optional] Location of the database
iosDatabaseLocation
String
[optional] iOS Database Location. Available options are:
Return Value
Promise
Example
An example of opening a database access handle object with a default or a specified location:
// use the default location
var database = window.sqlitePlugin.openDatabase({name: 'my.db', location: 'default'});
// use a specified location for iOS only
var database = window.sqlitePlugin.openDatabase({name: 'my.db', iosDatabaseLocation: 'Library'});
The successcb
and errorcb
callback parameters are optional but can be extremely helpful in case anything goes wrong. For example:
window.sqlitePlugin.openDatabase({name: 'my.db', location: 'default'}, function(db) {
db.transaction(function(tx) {
// ...
}, function(err) {
console.log('Open database ERROR: ' + JSON.stringify(err));
});
});
SQL transactions
Execute SQL on the opened database.
database.executeSql(SQL_STATEMENT: String, [] , [successCallback], [errorCallback]);
Return Value
Promise
Example
database.transaction(function(transaction) {
transaction.executeSql('SELECT count(*) AS recordCount FROM SampleTable', [], function(ignored, resultSet) {
$('#result').text('RECORD COUNT: ' + resultSet.rows.item(0).recordCount);
});
}, function(error) {
alert('SELECT count error: ' + error.message);
});
database.transaction(function(transaction) {
transaction.executeSql('DELETE FROM SampleTable');
}, function(error) {
alert('DELETE error: ' + error.message);
}, function() {
alert('DELETE OK');
});
Deleting a database
Delete a database access handle object.
window.sqlitePlugin.deleteDatabase({name: String, [location: String], [iosDatabaseLocation: String]}, [successCallback], [errorCallback]);
Parameter
Name
Type
Description
name
String
Name of the database
location
String
[optional] Location of the database
iosDatabaseLocation
String
[optional] iOS Database Location. Available options are:
Return Value
Promise
Example
// use the default location
window.sqlitePlugin.deleteDatabase({name: 'my.db', location: 'default'}, successcb, errorcb);
// use a specified location for iOS only
window.sqlitePlugin.deleteDatabase({name: 'my.db', iosDatabaseLocation: 'Library'});
Last updated
Was this helpful?