Phonegap Push Plugin
Deprecated
This Cordova plugin is used to register and receive push notifications.
Repository: https://github.com/phonegap/phonegap-plugin-push
Plugin ID/package name:
phonegap-plugin-push
Tested version:
2.3.0
Demo
Import the Phonegap push demo to your Monaca account
Preparation for iOS
When working with push notifications for iOS, an APNs authentication key or an APNs certificate is required. This section will show you how to create a development APNs certificate for testing on an iOS debug build. For release build, a production APNs certificate is required.
In order to continue, you should have signed up to the Apple Developer Program. Follow the instructions below to create a development APNs certificate.
From the Apple Developer page, go to
Account
.Select Certificates, Identifiers & Profiles.

3. An app ID with push notifications enabled is required when creating an APNs certificate. Therefore, let’s create one. Under Identifiers
section, go to the App IDs
and click the âž• button in the upper right corner.
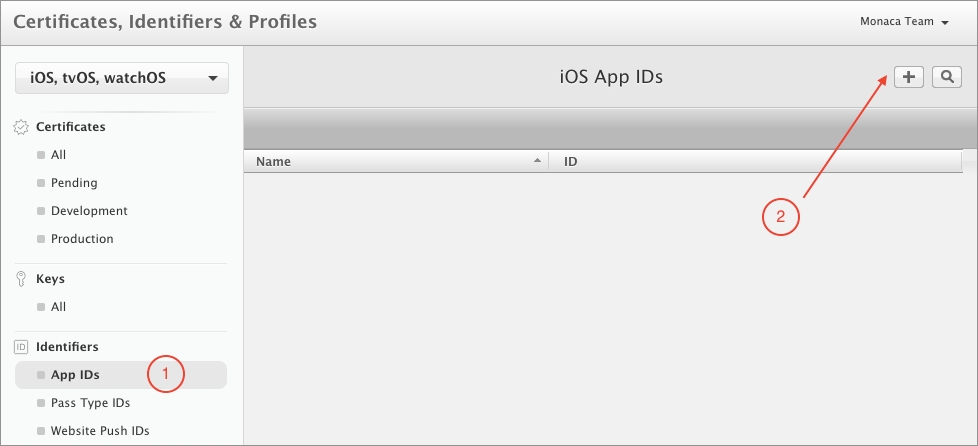
4. Fill in the app ID information:
App Description
: Input your app name here (e.g. Cordova Firebase Demo)Explicit ID
: Select this option because a Wildcard App ID is not allowed for push notifications. Input a unique identifier for your app (e.g. io.monaca.firebase).
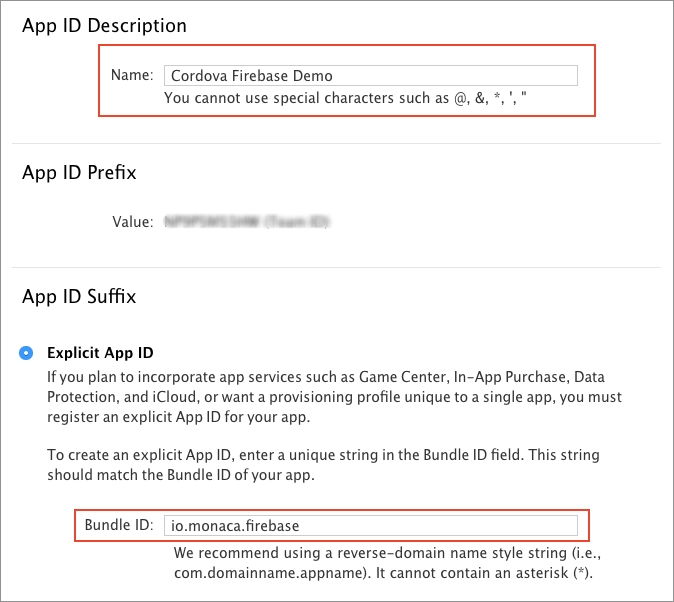
5. Scroll down to the App Services
section and tick the Push Notifications option. Then, click Continue.
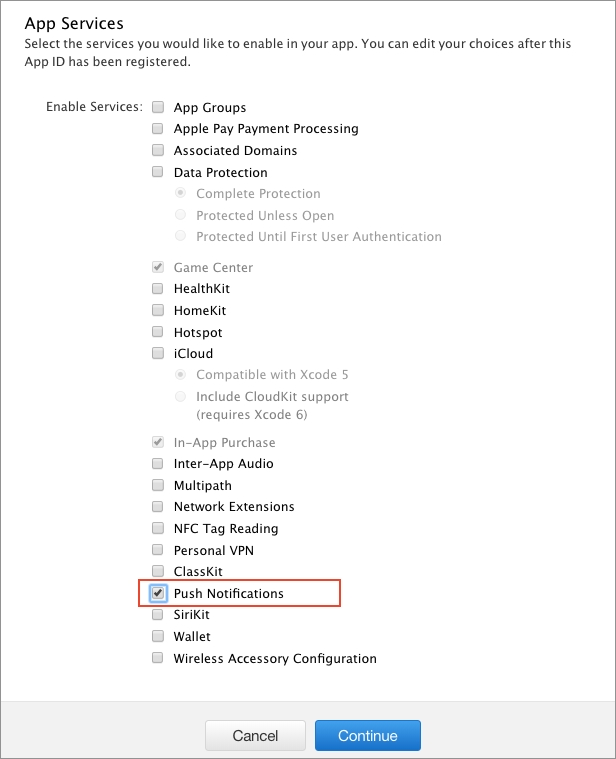
6. After that, you will be redirected to the Confirm your App ID
page. Click Register to complete the process.
7. Now, you should be back to the iOS App IDs page. Select the App ID you’ve just created and click Edit.
8. Scroll down to the Push Notifications
section, click Create Cerficate under Development SSL Certificate
.
9. Click Continue.
10. Click Choose File to browse your CSR file. You can get this file from the Monaca Cloud IDE by going to Config → iOS Build Settings. Then, click Generate Key and CSR. After creating the CRS file, download it by clicking the Export button.
11. Next, click Continue again. When the certificate is ready, you can download it. Save the file.
12. Upload the downloaded certificate by clicking the Upload Certificate button in the iOS Build Settings.
13. Click the Export icon of the target certificate displayed in the Certificates registered in Monaca to export the p12
file. You will need it for the Firebase configuration in the upcoming section.
Configuring Firebase for push notifications
Go to the Firebase Console.
Sign in with your Google account to enter the console.
Click Add project.

4. Fill in the project information and click CREATE PROJECT. Then, you will be redirected to the Project Overview
page.
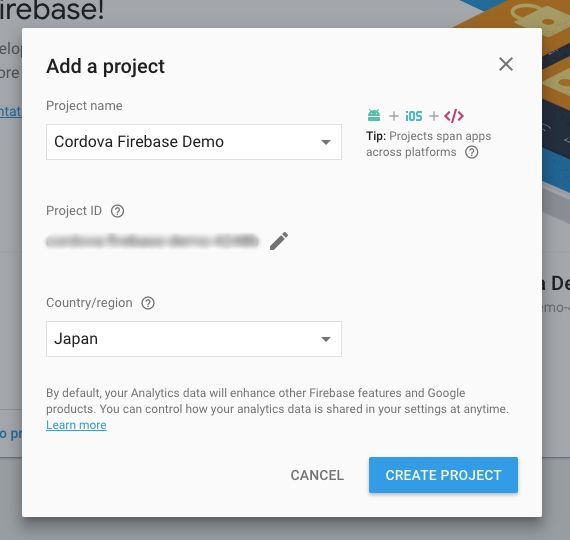
After project creation, we can configure the push notification project for a specific platform.
For iOS
Go to the
Project settings
.

2. Under the General section, click ADD APP and select iOS.
3. Enter your iOS Bundle ID (You can find that by going to Config → iOS App Settings). Then, click REGISTER APP.
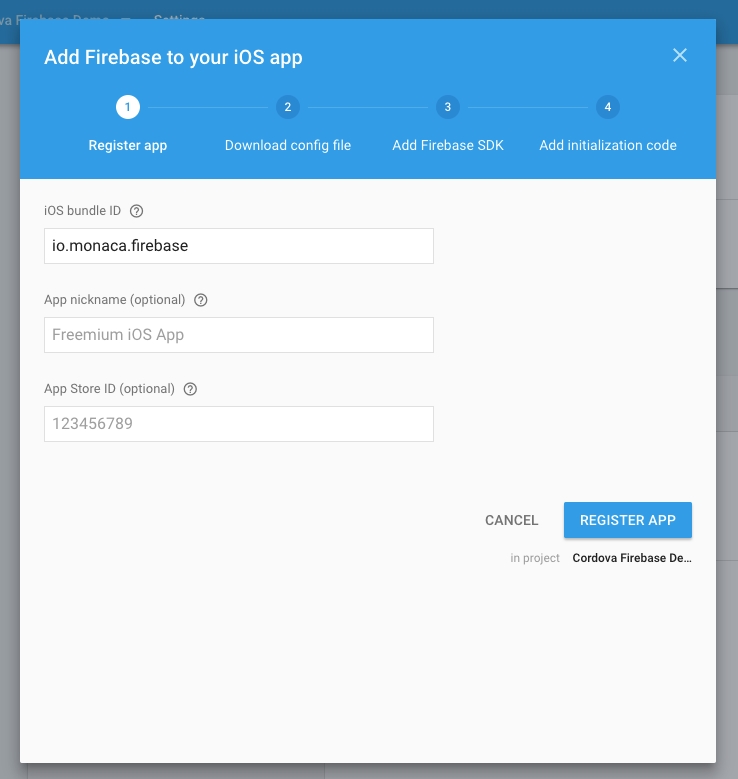
4. Download the GoogleService-Info.plist
file and place it in the root folder of your project. After that, click CONTINUE.
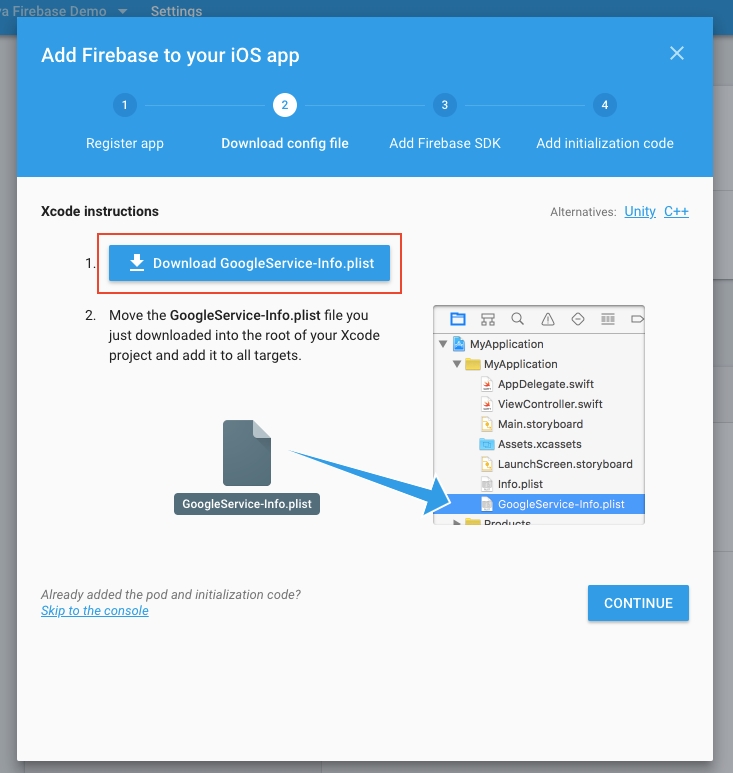
5. You will see the instructions on how to add the Firebase SDK to our project. However, we are not developing the app natively so we can skip this step. Just click CONTINUE to proceed. Then, click FINISH to complete the configuration. After that, you should see your iOS app in the Firebase overview page.
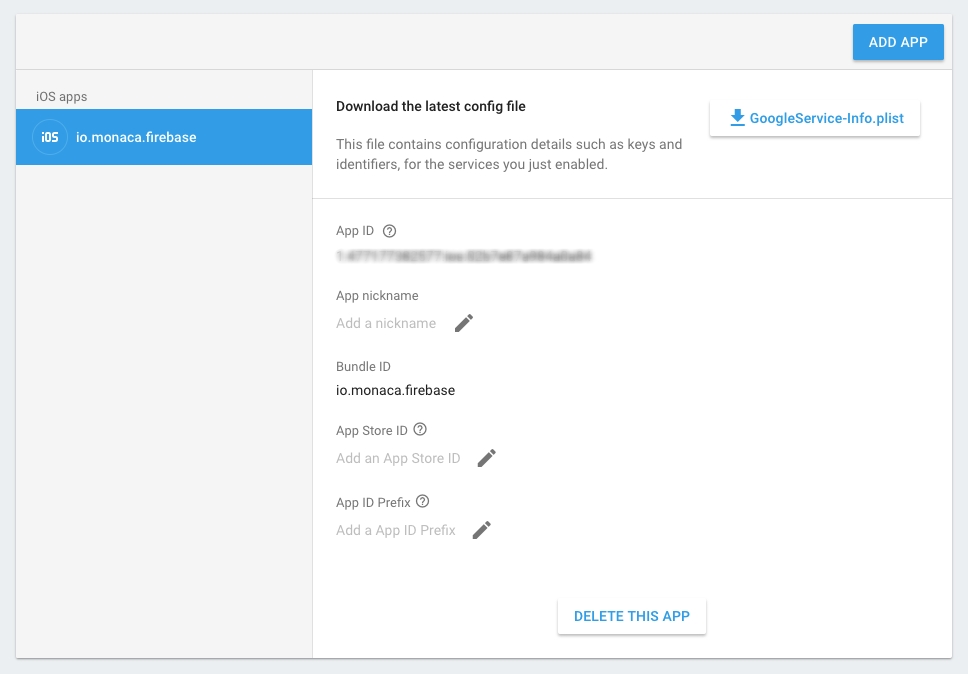
6. Now, it’s time to add the APNs certificates to the project. Go to the Project settings
and select CLOUD MESSAGING.
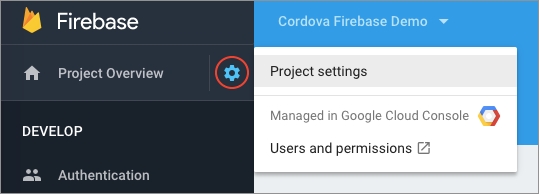
7. Scroll down to the iOS app configuration section and upload the APNs authentication key or APNs certificates.
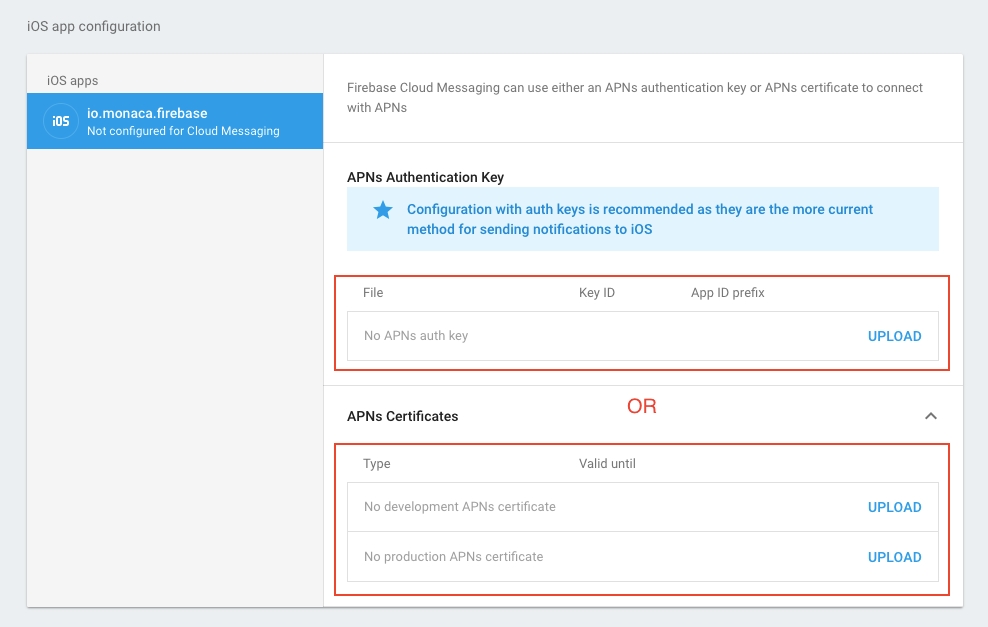
That’s it! You are done with iOS configuration.
For Android
Go to
Project settings
.

2. Under the General
section, click ADD APP and select Android.
3. Enter your package name (You can find that by going to Config → Android App Settings). Then, click REGISTER APP.
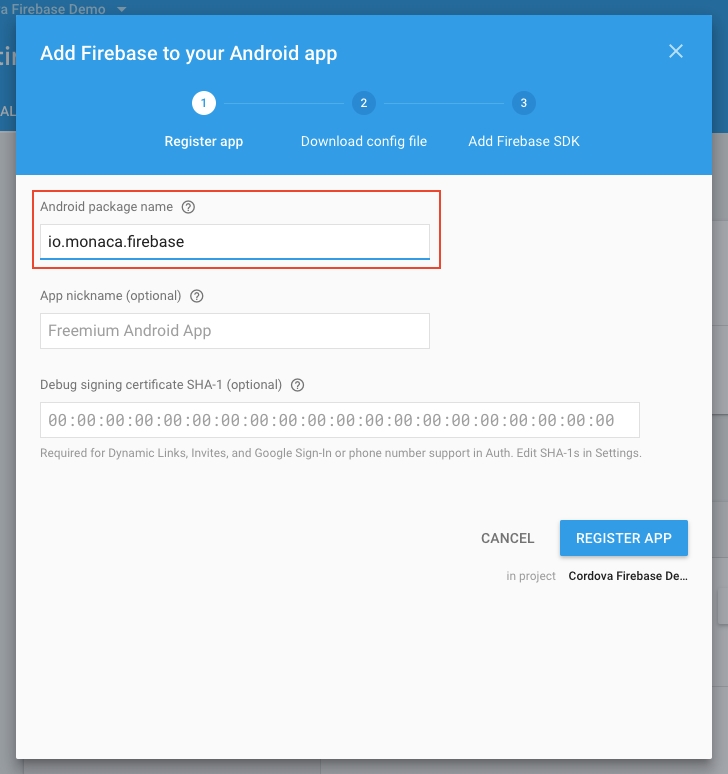
4. Download the google-services.json
file and place it in the root folder of your project. After that, click CONTINUE.
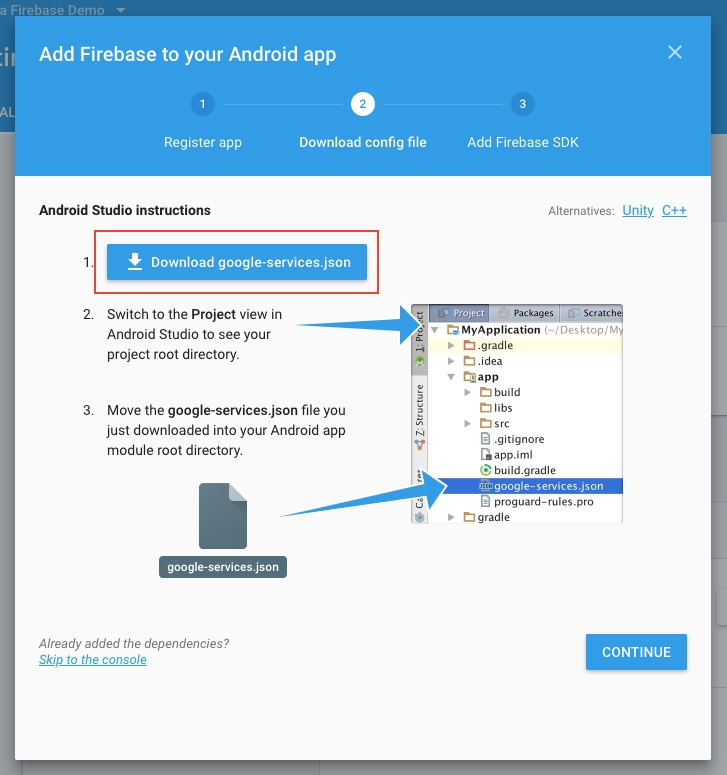
5. You will see instructions on how to add the Firebase SDK into our project. However, we are not developing the app natively so we can skip this step. Just click FINISH to complete the configuration. After that, you should see your Android app in the Firebase overview page.
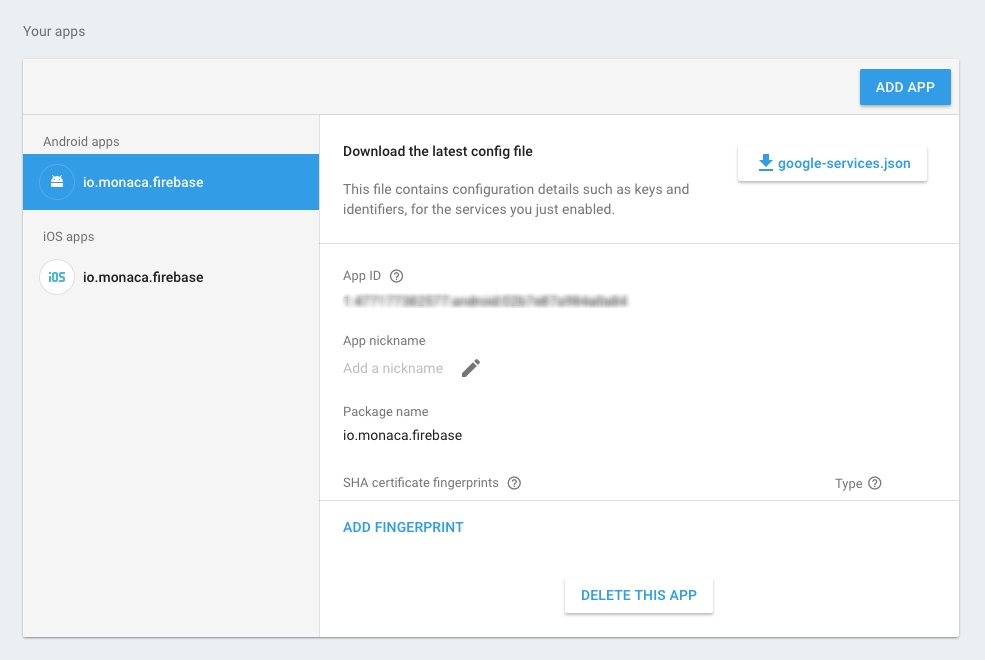
Adding the plugin to the Monaca IDE
From the IDE menu, go to Config → Manage Cordova Plugins .
Click the Import Cordova Plugin button. Then, you can choose to import the plugin using a ZIP file or a URL/package name.
Usage
After importing the plugin to the project, you can initialize the push notifications. Please make sure to call the plugin API after the Cordova is loaded. For example:
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
// Notification initialization
push = PushNotification.init({
ios: {
badge: true
}
});
// Checking notification permission
$interval(checkNotifPermiss, 500);
// Updating App Icon Badge Number
$interval(updateAIBN, 500);
// When receiving a push notification
push.on('notification', data => {
receiveNotifCB(data);
});
}
API references
This section describes some of the main functions used in our demo. For complete API references, please refer to the repository.
init()
Initialize push notifications.
PushNotification.init(options)
Parameter
Name
Type
Description
options
Object
An object describing relevant specific options for all target platforms.
iOS
Attribute
Type
Default
Description
ios.voip
Boolean
false
[optional] If set to true
, the device will be set up to receive VoIP Push notifications and the other options will be ignored since VoIP notifications are silent notifications that should be handled in the notification
event.
ios.alert
Boolean
false
[optional] If set to true
, the device shows an alert on receipt of notification.*
ios.badge
Boolean
false
[optional] If set to true
, the device sets the badge number on receipt of notification.*
ios.sound
Boolean
false
[optional] If set to true
, the device plays a sound on receipt of notification.*
ios.clearBadge
Boolean
false
[optional] If set to true
, the badge will be cleared on app startup.
ios.categories
Object
{}
[optional] The data required in order to enabled Action Buttons for iOS. See Action Buttons on iOS for more details.
*
: Please note that the value you set this option to the first time you call the init method will be how the application always acts. Once this is set programmatically in the init method it can only be changed manually by the user in . This is normal iOS behaviour.
Android
Attribute
Type
Default
Description
android.icon
String
[Optional] The name of a drawable resource to use as the small-icon. The name should not include the extension.
android.iconColor
String
[Optional] Sets the background color of the small icon on Android 5.0 and greater. [Supported Formats](http://developer.android.com/reference/android/graphics/Color.html#parseColor(java.lang.String))
android.sound
Boolean
true
[Optional] If set to true
, it plays the sound specified in the push data or the default system sound.
android.vibrate
Boolean
true
[Optional] If set to true
the device vibrates on receipt of notification.
android.clearBadge
Boolean
false
[Optional] If set to true
the icon badge will be cleared on init and before push messages are processed.
android.clearNotifications
Boolean
true
[Optional] If set to true
, the app clears all pending notifications when it is closed.
android.forceShow
Boolean
false
[Optional] Controls the behavior of the notification when app is in foreground. If true and app is in foreground, it will show a notification in the notification drawer, the same way as when the app is in background (and on('notification') callback will be called only when the user clicks the notification). When false and app is in foreground, the on('notification') callback will be called immediately.
android.topics
Array
[]
[Optional] If the array contains one or more strings each string will be used to subscribe to a FcmPubSub topic.
android.messageKey
String
message
[Optional] The key to search for text of notification
android.titleKey
String
title
[Optional] The key to search for title of notification
Return Value
pushObject
Example
const push = PushNotification.init({
android: {},
browser: {
pushServiceURL: 'http://push.api.phonegap.com/v1/push'
},
ios: {
alert: 'true',
badge: true,
sound: 'false'
},
windows: {}
});
hasPermission()
Check whether the push notification permission has been granted on the device.
PushNotification.hasPermission(successHandler)
Parameter
Name
Type
Description
successHandler
Function
Is called when the api successfully retrieves the details on the permission.
Callback Parameters
successHandler
Name
Type
Description
data.isEnabled
Boolean
Whether the permission for push notifications has been granted.
Return Value
Promise
Example
PushNotification.hasPermission(data => {
if (data.isEnabled) {
console.log('isEnabled');
}
});
getApplicationIconBadgeNumber()
Get the current badge count visible when the app is not running
push.getApplicationIconBadgeNumber(successHandler, errorHandler)
Parameter
Name
Type
Description
successHandler
Function
Is called when the api successfully retrieves the icon badge number.
errorHandler
Function
Is called when the api encounters an error while trying to retrieve the icon badge number.
Callback Parameters
successHandler
Name
Type
Description
n
Number
An integer which is the current badge count
Return Value
Promise
Example
push.getApplicationIconBadgeNumber(
n => {
$scope.aibn = n;
},
() => {
console.log('Error getting the number');
}
);
setApplicationIconBadgeNumber()
Set the badge count visible when the app is not running.
push.setApplicationIconBadgeNumber(successHandler, errorHandler, count)
Parameter
Name
Type
Description
successHandler
Function
Is called when the api successfully retrieves the icon badge number.
errorHandler
Function
Is called when the api encounters an error while trying to retrieve the icon badge number.
count
Number
Indicates what number should show up in the badge. Passing 0
will clear the badge. Each notification
event contains a data.count value which can be used to set the badge to correct number.
Return Value
Promise
Example
push.setApplicationIconBadgeNumber(
() => {
alert("Successfully setting the badge number!");
},
() => {
alert("Fail to set the badge number. Something went wrong...");
},
badgeNum
);
push.on()
The event notification
will be triggered each time a push notification is received by a 3rd party push service on the device.
push.on('notification', callback)
Callback Parameters
Name
Type
Description
data.message
String
The text of the push message sent from the 3rd party service
data.title
String
[optional] The title of the push message sent from the 3rd party service
data.count
String
The number of messages to be displayed in the badge in iOS/Android or message count in the notification shade in Android. For windows, it represents the value in the badge notification which could be a number or a status glyph.
data.sound
String
The name of the sound file to be played upon receipt of the notification
data.image
String
The path of the image file to be displayed in the notification
data.launchArgs
String
The args to be passed to the application on launch from push notification. This works when notification is received in background. (Windows Only)
data.additionalData
Object
[optional] A collection of data sent by the 3rd party push service that does not fit in the above properties
data.additionalData.foreground
Boolean
Whether the notification was received while the app was in the foreground
data.additionalData.coldstart
Boolean
Will be true if the application is started by clicking on the push notification, false if the app is already started.
data.additionalData.dismissed
Boolean
Is set to true if the notification was dismissed by the user
Return Value
Promise
Example
push.on('notification', data => {
console.log(data.message);
console.log(data.title);
console.log(data.count);
console.log(data.sound);
console.log(data.image);
console.log(data.additionalData);
});
Last updated
Was this helpful?