Cordova Google Analytics Plugin
This Cordova plugin is used to connect to Google's native Universal Analytics SDK.
Plugin ID/package name:
cordova-plugin-google-analytics
Tested version: 1.8.6
Demo
Import the Google Analytics plugin demo to your Monaca account
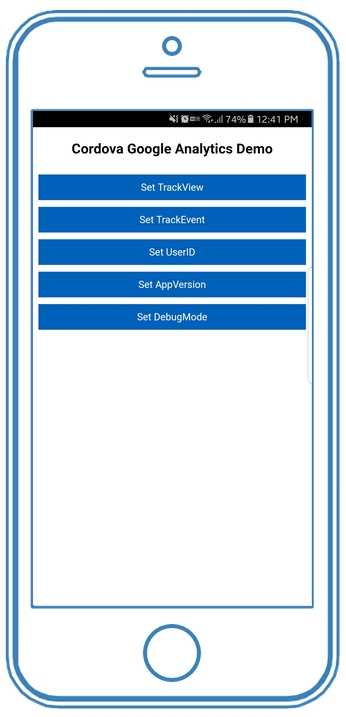
Enable the plugin in the Monaca IDE
From the IDE menu, go to Config → Manage Cordova Plugins .
Click the Import Cordova Plugin button. Then, you can choose to import the plugin using a ZIP file or a URL/package name.
Usage
After importing the plugin to your project, you can start by initializing your tracking ID. Make sure to call the plugin API after the Cordova is loaded.
//Replace your app tracking id here
var trackingID="YOUR_APP_TRACKING_ID";
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady(){
console.log('Google analytics is ready now');
window.ga.startTrackerWithId(trackingID);
}
API references
This section describes some of the main functions used in our demo. For complete API references, please refer to the repository.
startTrackerWithId()
Sets up the analytics tracker.
window.ga.startTrackerWithId(trackingId, [interval]);
Parameter
Name
Type
Description
trackingId
String
Your Google Analytics mobile app property
interval
Number
[optional] The dispatch period in seconds (default: 30
)
Return Value
Promise
Example
window.ga.startTrackerWithId('UA-XXXX-YY', 30);
trackView()
Tracks the screen.
window.ga.trackView(title, campaignUrl, [newSession]);
Parameter
Name
Type
Description
title
String
Screen title
campaignUrl
String
Campaign url for measuring referrals
newSession
Boolean
[optional] Set to true
to create a new session
Return Value
Promise
Example
//To track a Screen (PageView):
window.ga.trackView('Screen Title')
//To track a Screen (PageView) w/ campaign details:
window.ga.trackView('Screen Title', 'my-scheme://content/1111?utm_source=google&utm_campaign=my-campaign')
//To track a Screen (PageView) and create a new session:
window.ga.trackView('Screen Title', '', true)
trackEvent()
Tracks an event.
window.ga.trackEvent(category, action, [label], [value], [newSession])
Parameter
Name
Type
Description
category
String
Event category (e.g. 'Video')
action
String
Action type (e.g. 'play')
label
String
[optional] Event label (e.g. 'Fall Campaign')
value
Number
[optional] A numeric value associated with the event (e.g. 42)
newSession
Boolean
[optional] Set to true
to create a new session
Return Value
Promise
Example
//To track an Event
window.ga.trackEvent('Videos', 'play', 'Fall Campaign', 42)
//To track an Event and create a new session:
window.ga.trackEvent('Videos', 'play', 'Fall Campaign', 42, true)
setUserId()
Sets a user id.
window.ga.setUserId(id);
Parameter
Name
Type
Description
id
String
A unique identifier, associated with a particular user, must be sent with every hit
Return Value
Promise
Example
//user ID for testing purpose
var myUserId="35009a79-1a05-49d7-b876-2b884d0f825b";
window.ga.setUserId(myUserId);
setAppVersion()
Sets a specific app version.
window.ga.setAppVersion(appVersion)
Parameter
Name
Type
Description
appVersion
String
App version
Return Value
Promise
Example
window.ga.setAppVersion('1.33.7');
debugMode()
Enables verbose logging.
window.ga.debugMode()
Return Value
Promise
Example
window.ga.debugMode();
Last updated
Was this helpful?